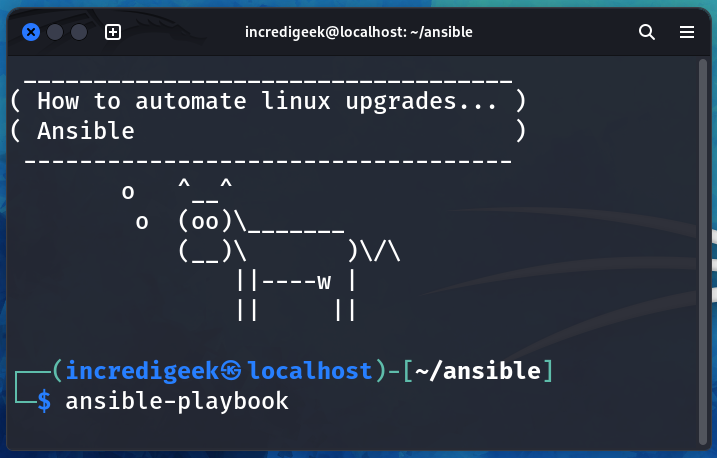
The three steps to update a machine with Ansible
- Create Ansible Inventory/Hosts file
- Create Playbook
- Run Playbook
Create Inventory
The first thing we need to do is create an inventory file. This will contain a list of our servers along with the credentials.
touch hosts.txt
Now let’s encrypt the file with Ansible Vault.
ansible-vault encrypt hosts.txt
The file is now encrypted. To edit the file, we need to use `ansible-vault edit`.
If you want to, you can configure the hosts.txt file and then encrypt it when you are finished.
ansible-vault edit hosts.txt
Now add some hosts. In this example we add the local Kali machine, because why not. If you have Ubuntu servers, replace debian with ubuntu.
[debian] kali ansible_host=127.0.0.1 ansible_ssh_user=kali ansible_ssh_port=22 ansible_ssh_password='kali pass' ansible_become_pass='kali sudo pass'
Add as many hosts as you need. For sake of simplicity, we are only adding one, and it is our localhost.
Create Playbook
Create a new playbook.
vi debian_update.yml
Put the following into the playbook. Edit as desired. Change hosts to match the above hosts in the inventory/hosts file.
---
- name: OS update
hosts: debian
gather_facts: yes
become: yes
tasks:
- name: dist-upgrade
ansible.builtin.apt:
upgrade: dist
update_cache: yes
register: upgrade_result
- name: Check if a reboot is required
ansible.builtin.stat:
path: /var/run/reboot-required
get_checksum: no
register: reboot_required_file
- name: Reboot the server (if required).
ansible.builtin.reboot:
when: reboot_required_file.stat.exists
register: reboot_result
- name: Remove unneeded dependencies
ansible.builtin.apt:
autoremove: yes
register: autoremove_result
- name: Print errors if upgrade failed
ansible.builtin.debug:
msg: |
Upgrade Result: {{ upgrade_result }}
Reboot Result: {{ reboot_result }}
Autoremove Result: {{ autoremove_result }}
A couple of notes
- On the 3rd line it defines which group to run this playbook against. In this case debian.
- This will check if a reboot is needed and reboot the machine. Reboots are usually needed when the kernel is updated
- The 5th line contains `become: yes` this means that the playbook will use sudo. You can specify the sudo password in the hosts file `ansible_become_pass=sudopass` or with the -k or –ask-become options
- The update and reboot are natively built into Ansible. Hence the ansible.builtin.
Run Playbook
Now that we have our inventory and playbook, we can upgrade our machines.
ansible-playbook debian_update.yml -i hosts.ini --ask-vault-password
Tip! If you have not specified a “ansible_ask_become” password (that is the sudo password), you can specify it with the -k or –ask-become options.