You’ll need a touch screen to test this out. Easiest way is to use the Unity Remote 5 app. It allows you to use your Android device as a touch screen input.
Start by creating a new empty C# script named “TouchScript” and paste in the following code.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class TouchScript : MonoBehaviour {
public GameObject bullet;
void Start () {
}
void Update () {
Touch myTouch = Input.GetTouch (0);
for(int i =0; i < Input.touchCount; i++)
if (myTouch.phase == TouchPhase.Began) {
{
Debug.Log ("Touch Position" + myTouch.position);
SpawnBullet (myTouch);
}
}
}
void SpawnBullet(Touch fireTouch) {
Vector3 touchPos = Camera.main.ScreenToWorldPoint (fireTouch.position);
touchPos.z = 1; // Puts the z coordinates at 1 so it is visible to the camera.
Debug.Log("Vector3 Pos" + touchPos);
Instantiate (bullet, touchPos, Quaternion.identity);
}
}
Now you’ll need to put your script on a game object. You should be able to drag and drop it on any game object, in this case the Main Camera “green”.
Next drag and drop the game object prefab to the script “red”. This tells the script which game object to spawn or instantiate when you touch the screen.
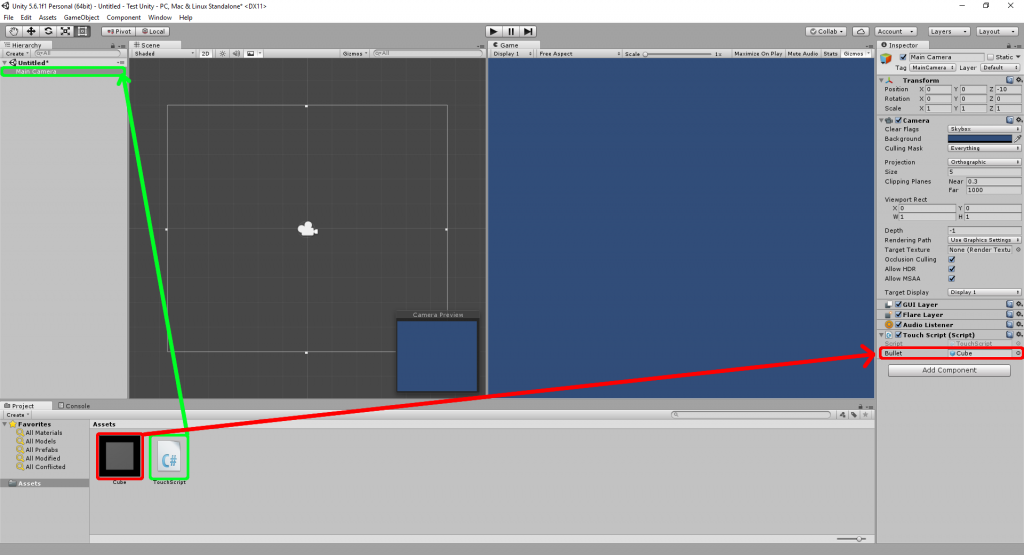
Run the scene. It should now create a game object where you touch on the screen.
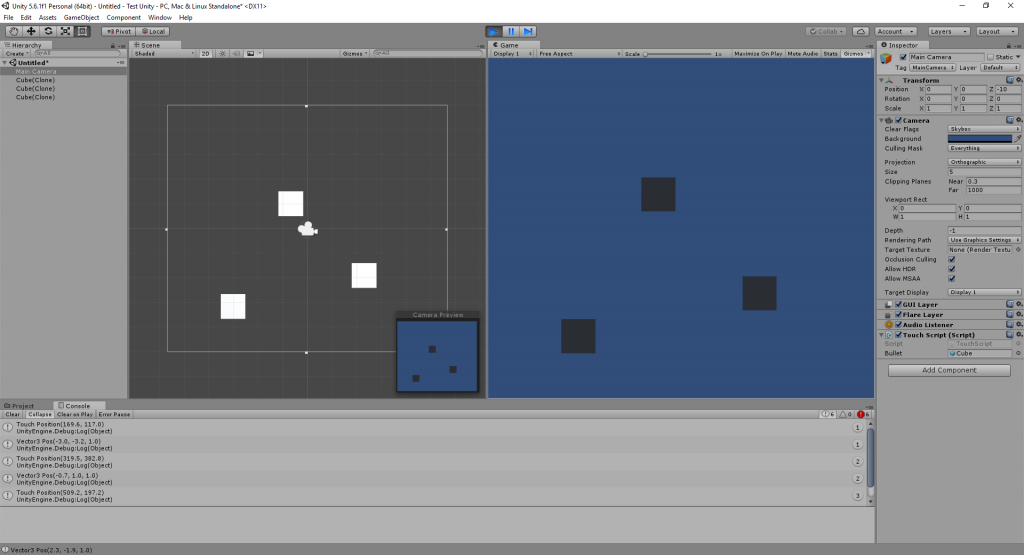