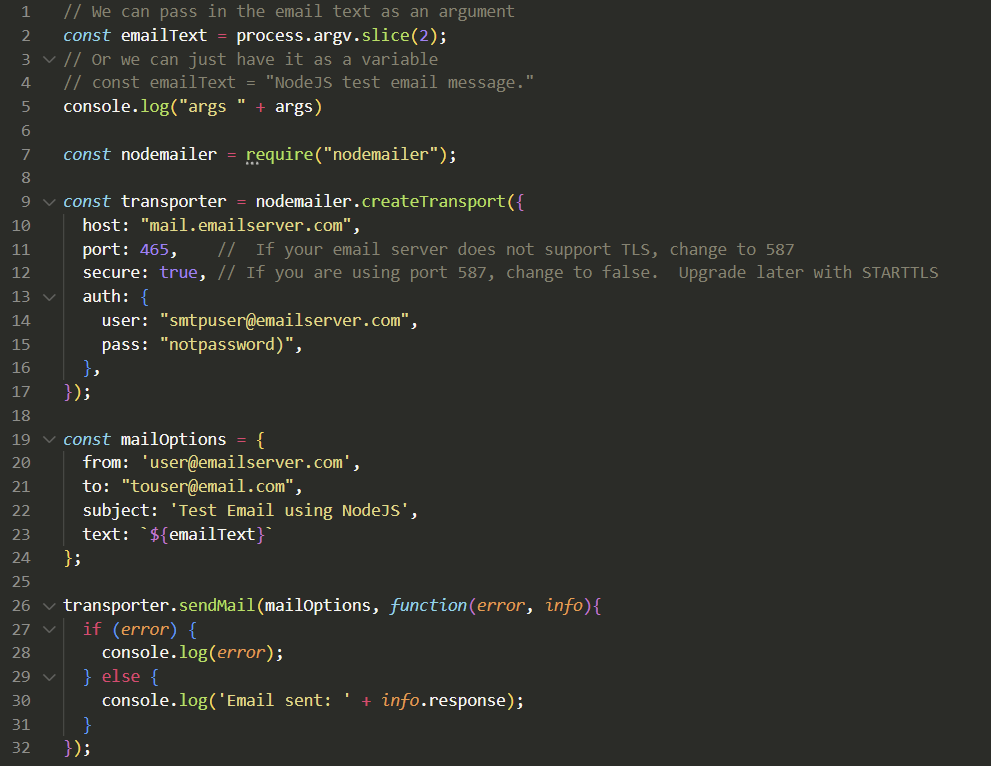
In this post, we will be using Node.JS and the nodemailer library to send email. We need to have an email account with an email provider to send email. Gmail or some other email provider should work.
Prerequisites
First lets install some tools
sudo apt install nodejs npm
Now lets install nodemailer
npm install nodemailer
Writing the Code to Send Email
Now that we have nodemailer installed, we can write or copy our code. Create a file called maill.js and make it look similar to the following.
// We can pass in the email text as an argument
const emailText = process.argv.slice(2);
// Or we can just have it as a variable
// const emailText = "NodeJS test email message."
console.log("args " + args)
const nodemailer = require("nodemailer");
const transporter = nodemailer.createTransport({
host: "mail.emailserver.com",
port: 465, // If your email server does not support TLS, change to 587
secure: true, // If you are using port 587, change to false. Upgrade later with STARTTLS
auth: {
user: "smtpuser@emailserver.com",
pass: "notpassword)",
},
});
const mailOptions = {
from: 'user@emailserver.com',
to: "touser@email.com",
subject: 'Test Email using NodeJS',
text: `${emailText}`
};
transporter.sendMail(mailOptions, function(error, info){
if (error) {
console.log(error);
} else {
console.log('Email sent: ' + info.response);
}
});
Update the following variables
- host: to your host email server
- user: to the email user that is sending email. It should have an account on the email server
- pass: password for your email user that is sending the email
- from: email address that is sending the email
- to: email account(s) you are sending email to
- subject: subject of your email
Now we can proceed to send email
Sending Email
We can now run the code by saving our file and running it directly with NodeJS
nodejs ./mail.js "This is the body text for the email"
Hit Return and look for the email. If something went wrong, it should throw an error.
You can change the emailText variable if you would rather have the message body inside the code.
Code Explanation and Notes
A little explanation on the code.
The second line “const emailText = process.argv.slice(2);” is used to pass in a command line argument to use as the text for the body of the email. You can delete the line and uncomment line 4 if you would rather use a variable inside the code.
Your email server should support using SSL/TLS on port 465. If it does not, you may need to use STARTTLS which uses port 587, and then set secure to false. STARTTLS should upgrade the connection to be encrypted. But it’s opportunistic. You can read more about STARTTLS, SSL/TLS here https://mailtrap.io/blog/starttls-ssl-tls/
You can change the “to: ” in the mailOptions object to an array of email addresses to send the email to multiple people at once.
to: ["email1@email.com", "email2@email.com", "etc"],