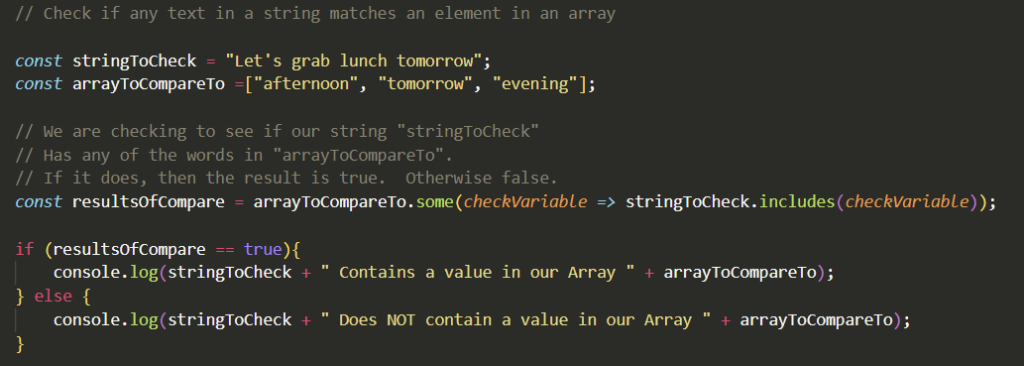
In the following code we will be checking a string and check if any of the words in the string match some or any elements in an array.
We can imagine that our “stringToCheck” variable is an email or message response. We want to know if it contains a mention to afternoon, tomorrow, or evening. Presumably so we can automate something. Note that the matches are case sensitive.
// Check if any text in a string matches an element in an array
const stringToCheck = "Let's grab lunch tomorrow";
const arrayToCompareTo =["afternoon", "tomorrow", "evening"];
// We are checking to see if our string "stringToCheck"
// Has any of the words in "arrayToCompareTo".
// If it does, then the result is true. Otherwise false.
const resultsOfCompare = arrayToCompareTo.some(checkVariable => stringToCheck.includes(checkVariable));
if (resultsOfCompare == true){
console.log(stringToCheck + " Contains a value in our Array " + arrayToCompareTo);
} else {
console.log(stringToCheck + " Does NOT contain a value in our Array " + arrayToCompareTo);
}
More examples and ways to do it are available at the following link.