The Spread and Rest operators i.e. the three dots (…) can be used to make code cleaner and more concise.
Difference between Spread and Rest
Spread: Works on elements on the right side of the = operator, and breaks them out into individual elements.
Rest: Works on the left hand side of the = operator, and compresses them into an array.
Using Spread to Iterate over Arrays
Spread works on iterables like strings, arrays, maps and sets.
The spread operator operates similar to taking all the elements out of an array and operating on them or writing them to a new array. Say for instance we have an array of computers and we want to log each element to the console.
const computersA = ['Acer', 'Apple', 'ASUS']
We can log each element by running
console.log(computersA[0], computersA[1], computersA[2])
Or we can use the spread operator
console.log(...computersA)
The output is the same.
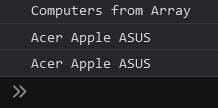
Joining Arrays
We can also use the spread operator to join two arrays together. Say we have two arrays
const computersA = ['Acer', 'Apple', 'ASUS']
const computersB = ['HP', 'Dell', 'Lenovo']
And we want to concatenate them together. We can do that simply by
const computerAll = [...computersA, ...computersB]
Rest Example
Rest is simply the opposite of spread. Spread take an item like an array and expands it out into elements we can use. Rest takes elements and packs them into an array. This can be extremely helpful if we want to pass in an unknown amount of elements into a function for processing.
const computersA = ['Acer', 'Apple', 'ASUS']
function writeToLog (...arr) {
for (const element of arr) {
console.log(element)
}
}
Now we can call the function with as many elements in the array and they will all get logged to the console.
writeToLog('Razer', 'Alienware', 'Legion')
We could also use both the Spread and Rest functions
const gamingLaptops = ['Razer', 'Alienware', 'Legion']
writeToLog(...gamingLaptops)
Now as we add more laptops to the gamingLaptops array, the function will automatically process the line and write to console.
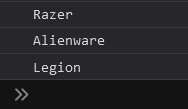
https://www.freecodecamp.org/news/three-dots-operator-in-javascript/